Python scripting tutorial
Python is a programming language, very simple to use and very fast to learn. It is open-source, multi-platform, and can be used alone for a wide array of things, from programming simple shell scripts to very complex programs. But one of its most widespread uses is as a scripting language, since it is easy to embed in other applications. That's exactly how it is used inside FreeCAD. From the python console, or from your custom scripts, you can pilot FreeCAD, and make it perform very complex actions for which there is still no graphical user interface tool.
For example, from a python script, you can:
- create new objects
- modify existing objects
- modify the 3D representation of those objects
- modify the FreeCAD interface
There are also several different ways to use python in FreeCAD:
- From the FreeCAD python interpreter, where you can issue simple commands like in a "command line"-style interface
- From macros, which are a convenient way to quickly add a missing tool to the FreeCAD interface
- From external scripts, which can be used to program much more complex things. like entire Workbenches.
In this tutorial, we'll work on a couple of simple examples to get you started, but there is also much mode documentation about python scripting available on this wiki. If you are totally new to python and want to understand how it works, we also have a basic introduction to python.
Writing python code
There are two easy ways to write python code in FreeCAD: From the python console (available from the View -> Views -> Python console menu) or from the Macro editor (Tools -> Macros). In the console, you write python commands one by one, which are executed when you press return, while the macros can contain a more complex script made of several lines, which is executed only when the macro is executed.
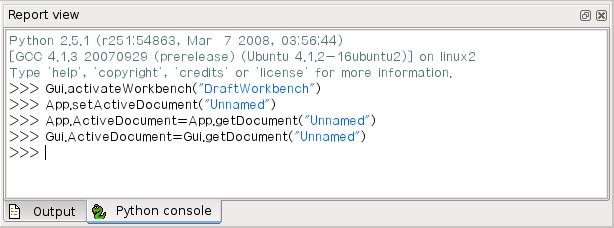
In this tutorial, you will be able to use both methods, either by copying/pasting each line one by one in the python console and pressing Return after each line, or by copying/pasting the entire code in a new Macro window.
Exploring FreeCAD
Let's start by creating a new empty document:
doc = FreeCAD.newDocument()
If you type this in the FreeCAD python console, you will notice that as soon as you type "FreeCAD.", a windows pops up, allowing to quickly autocomplete the rest of your line. Even better, each entry in the autocomplete list has a tooltip explaining what it does. This makes it very easy to explore the functionality available. Before choosing "newDocument", have a look at the other options available.
Now our new document will be created. This is similar to pressing the "new document" button on the toolbar. In fact, most buttons in FreeCAD do nothing else than executing a line or two of python code. Even better, you can set an option in Edit -> Preferences -> General -> Macro to "show script commands in python console". This will print in the console all python code executed when you press buttons. Very useful to learn how to reproduce actions in python.
Now let's get back to our document. Let's see what we can do with it:
doc.
Explore the available options. Usually names that begin with a capital letter are attributes, they contain a value, while names that begin with small letter are functions (also called methods), they "do something". Names that begin with an underscore are usually there for the internal working of the module, and you shouldn't care about them. Let's use one of the methods to add a new object to our document:
box = doc.addObject("Part::Box","myBox")
Nothing happens. Why? Because FreeCAD is made for the big picture. One day, it will work with hundreds of complex objects, all depending one from another. Making a small change somewhere could have a big impact, you may need to recalculate the whole document, which could take a long time... For that reason, almost no command updates the scene automatically. You must do it manually:
doc.recompute()
See? Now our box appeared! Many of the buttons that add objects in FreeCAD actually do 2 things: add the object, and recompute. If you turned on the "show script commands in python console" option above, try now adding a sphere with the GUI button, you'll see the two lines of python code being executed one after the other.
What about the "Part::Box" will you ask? How can I know what other kind of objects can be added? It's all here:
doc.supportedTypes()
Now let's explore the contents of our box:
box.
You'll immediately see a couple of very interesting things such as:
box.Height
This will print the current height of our box. Now let's try to change that:
box.Height = 5
If you select your box with the mouse, you'll see that in the properties panel, in the "Data" tab, our "Height" property appears. All properties of a FreeCAD object that appear there (and also in the "View" tab, more about that later), are directly accessible by python too, by their names, like we did with the "Height" property. Try changing the other dimensions of that box.
A bit of theory
Now you must understand a couple of important concepts before we get further.
App and Gui
FreeCAD is made from the beginning to work as a command-line application, without its user interface. As a result, almost everything is separated between a "geometry" component and a "visual" component. When you work in command-line mode, the geometry part is present, but all the visual part is simply disabled. Almost any object in FreeCAD therefore is made of two parts, an Object and a ViewObject.
To illustrate the concept, see our cube object, the geometric properties of the cube, such as its dimensions, position, etc... are stored in the object, while its visual properties, such as its color, line thickness, etc... are stored in the viewobject. This corresponds to the "Data" and "View" tabs in the property window. The view object of an object is accessed like this:
v = box.ViewObject
Now you can also change the properties of the "View" tab:
v.Transparency = 80 v.hide() v.show()
Workbenches and Object types
Now you must surely wonder, what else than "Part::Box" can I do? The FreeCAD base application is more or less an empty container. Without its modules, it can do little more than creating new, empty documents. The true power of FreeCAD is in its faithful modules. Each of them adds not only new workbenches to the interface, but also new python commands and new object types. As a result, several different or even totally incompatible object types can coexist in the same document. The most important modules in FreeCAD, that we'll look at in this tutorial, are Part, Mesh, Sketcher or Draft.
Sketcher and Draft both use the Part module to handle their geometry. Mesh is totally independent, and its objects are of a different type that the 3 others.